Setting up a new workstation is easy. The only software required (docker, curl, git) is part of
most linux distributions.
Logged in with your regular user account (not root), open a terminal and execute:
sudo apt-get update && sudo apt-get install -y docker.io curl git ssh
sudo gpasswd -a $USER docker
git config --global user.name "John Doe"
git config --global user.email "johndoe@example.com"
git config --global credential.helper store
mkdir -p $HOME/Projects
ssh-keygen -t ed25519 && cat $HOME/.ssh/id_ed25519.pub
The last command will print your public ssh key:
ssh-ed25519 AAA..............some..more..chars...........J3jAPTge jondoe@example
Copy-n-paste the whole line and add it to your favorite development platform:
Finally install your favorite IDE. Logout and login again (to have changed user permissions applied). That’s it.
Container-based Software Development Kits
By using containers not only for deployment but also for development, there is no need
to install libraries on your workstation. Nor do you have to care about version
conflicts, environments, paths, etc.
No more buggy project setup documentation. git clone the project and run kickstart.
Everything else is done
Clone and start our example project
Change to your Projects-directory cd ~/Projects and clone infracamp’s demo project:
git clone https://github.com/infracamp/kickstart-demo.git. Change to the demo project directory
cd kickstart-demo and run kickstart.
After the container was downloaded and startet, you’ll see the bash prompt inside the container. You
can use git
, ssh
, bash history
like you do in your local shell. The project-directory is mounted
to /opt
inside the container:
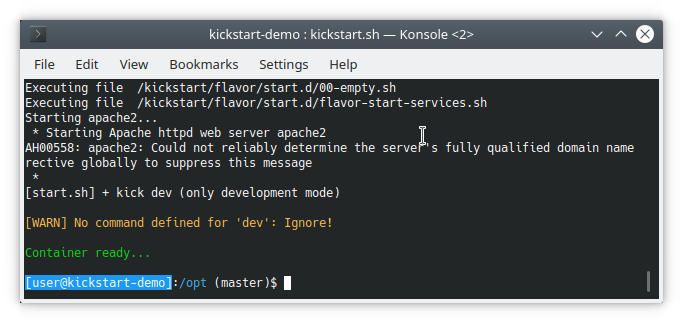
Open http://localhost:4300
in your browser.
What happened?
-
kickstart.sh evaluated the container configuration file .kick.yml
and runs the container infracamp/kickstart-flavor-gaia
specified in the from
section.
The container is running in development-mode: debugger, and other developer stuff is enabled.
-
The current project directory is mounted to /opt
directory inside the container and
user rights are adjusted to your local user rights. Environment variables are adjusted.
-
Inside the container, the starter script kick evaluates .kick.yml
and runs the
commands to install required stuff and setup the container.
-
To exit the container just type exit.
Run additional services in stack
To run additional services in development environment, just create a docker-compose like stack
file named .kick-stack.yml
in your projects root directory.
kickstart will automatically create a new network with
the name of your current project. Make sure to adjust the names
in your stack file:
.kick-stack.yml
version: "3"
services:
# This service will be available as: project_name_some_service
some_service:
image: some/image
networks:
- project_name ## <- Adjust these to your projects name
networks:
project_name: ## <- Adjust these to your projects name
external: true
Start your own project
.kick.yml - the container config file
The .kick.yml file is the central configuration file for
the container. It specifies the docker image to start. And is responsible
for configuring the container. Inside the container it is evaluated by the
kick command.
.kick.yml defines commands to be executed at build, dev, production
and test time. You can run these commands by running kick [command].
This has the advantage to test your scripts incremental inside the container
instead of rebuilding your container over and over again.
Defining commands and build stages in .kick.yml
version: 1
from: infracamp/kickstart-flavor-php:7.4
# Install packages via apt
packages: [wget, curl]
command:
build:
- "composer update"
- "npm install"
- "npm build"
dev:
- npm build --watch
run:
test:
- phpunit
Inside the container you can now execute e.g. the tests with kick test.
The Dockerfile
Keeping the container configuration inside .kick.yml helps reducing your
Dockerfile to only a few lines:
FROM nfra/kickstart-flavor-bare:1.0
ENV DEV_CONTAINER_NAME="your-project-name"
ADD / /opt
RUN ["bash", "-c", "chown -R user /opt"]
RUN ["/kickstart/run/entrypoint.sh", "build"]
ENTRYPOINT ["/kickstart/run/entrypoint.sh", "standalone"]
Make sure to adjust FROM
and DEV_CONTAINER_NAME
to the settings in
.kick.yml
Integration into CI/CD pipeline
This example shows how to integrate in .gitlab-cl.yml:
image: docker:stable
stages:
- build
services:
- docker:dind
before_script:
- apk update && apk add bash curl
- curl -o kickstart 'https://raw.githubusercontent.com/nfra-project/nfra-kickstart/master/dist/kickstart.sh' && chmod +x kickstart
latest:
stage: build
script:
- ./kickstart :test
- ./kickstart ci-build
only:
- master
- main
This will execute the unit-tests defined in command > test-section
and then build the container running command > build</kdb> command.
The command kickstart.sh ci-build will build the container using
Dockerfile and push the newly created container to gitlab’s registry.